This is a simple program that prints a few strings one after another in a loop, but it is randomized. This is very interesting. This goes to show it is very easy to do something like this.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 | #include <stdlib.h> #include <stdio.h> #include <time.h> char *unbase64(unsigned char *input, int length); const char* strings[] = {"This is string number one.","This is string number two.","This is string number three.","This is string number four."}; int main (void) { int lifetime; int k; srand((unsigned)time(NULL)); for (lifetime = 1; lifetime < 80; lifetime++) { k = rand() % 4; printf("%s\n", strings[k]); } return 0; } |
This is a simpler example of a loop, this is how to do a for loop in c.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 | #include "stdlib.h" #include "stdio.h" int main (void) { int lifetime; for (lifetime = 1; lifetime < 80; lifetime++) { printf("Wear Mask.\n"); printf("Stay 6ft apart.\n"); printf("Wash Hands\n"); printf("Get tested.\n\n"); } return 0; } |
Below is an example of very bad code, this is not even pseudocode, it is just bad. The example above will actually work.
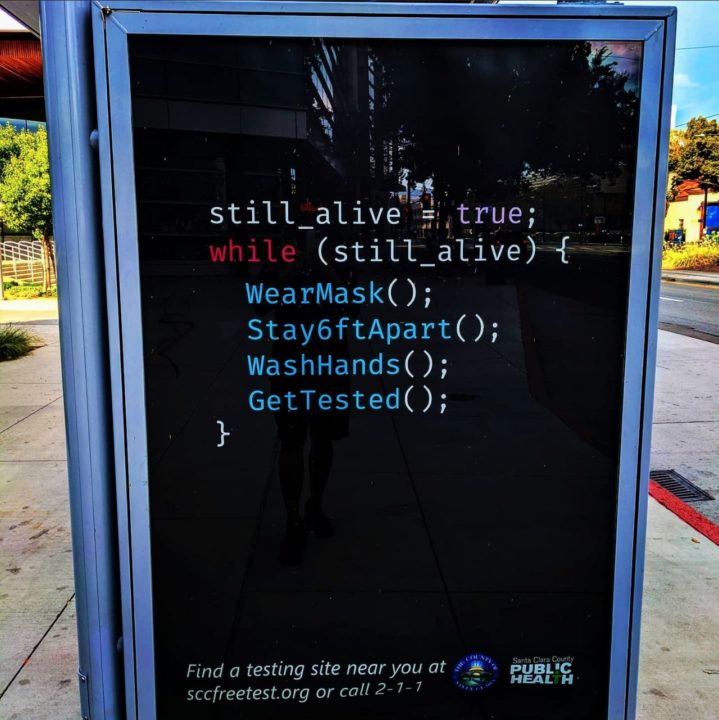
But this is what is found out in the wild. But these examples should really help you out when you are starting out in programming and want to know how to create a simple for loop. This is not very hard at all.