It is very easy to get the number of CPU cores using Powershell.
PS C:\Users\Intel i5> (Get-CimInstance Win32_ComputerSystem).NumberOfLogicalProcessors 12 |
But more information may be obtained this way.
PS C:\Users\Intel i5> Get-WmiObject -Class Win32_ComputerSystem -ComputerName. | Select-Object -Property SystemType,NumberOfLogicalProcessors,Name,Manufacturer,PrimaryOwnerName,Domain,TotalPhysicalMemory SystemType : x64-based PC NumberOfLogicalProcessors : 12 Name : DESKTOP-PF01IEE Manufacturer : ASUS PrimaryOwnerName : Intel i5 Domain : WORKGROUP TotalPhysicalMemory : 17026232320 |
And to get information about your GPU on Windows, use this one-liner.
PS C:\Users\Intel i5> Get-WmiObject win32_VideoController | Format-List Name,VideoModeDescription,DriverVersion,PNPDeviceID Name : NVIDIA GeForce RTX 2060 VideoModeDescription : 3440 x 1440 x 4294967296 colors DriverVersion : 30.0.14.9709 PNPDeviceID : PCI\VEN_10DE&DEV_1F08&SUBSYS_402B1458&REV_A1\4&38AB2860&0&0008 |
This very useful Powershell script will get your computer information in one go.
$SystemInfo = Get-CimInstance -ClassName Win32_ComputerSystem $CPUInfo = Get-CimInstance -ClassName Win32_Processor $MemoryInfo = Get-CimInstance -ClassName Win32_PhysicalMemory $OSInfo = Get-CimInstance -ClassName Win32_OperatingSystem Write-Host "Computer Name: $($SystemInfo.Name)" Write-Host "Manufacturer : $($SystemInfo.Manufacturer)" Write-Host "Model : $($SystemInfo.Model)" Write-Host "" Write-Host "CPU Information" foreach ($CPU in $CPUinfo) { Write-Host "- $($CPU.Name), Max Clock Speed: $($CPU.MaxClockSpeed) MHz" } Write-Host "" Write-Host "Memory Information (RAM)" foreach ($Memory in $Memoryinfo) { $CapacityGB = [math]::Round($memory.Capacity / 1GB, 2) Write-host "- Manufacturer: $($memory.Manufacturer), Size: ${CapacityGB} GB, Speed: $($memory.Speed) MHz" } Write-host "" # Display OS information. write-host 'Operating System:' write-host '- '$osinfo.caption,'('+$osinfo.+')' |
This will print very useful system information.
Below is the output.
PS C:\Users\Intel i5\Desktop> .\info.ps1 Computer Name: DESKTOP-PF01IEE Manufacturer : ASUS Model : System Product Name CPU Information - Intel(R) Core(TM) i5-10400F CPU @ 2.90GHz, Max Clock Speed: 2904 MHz Memory Information (RAM) - Manufacturer: Team Group Inc, Size: 8 GB, Speed: 3200 MHz - Manufacturer: Team Group Inc, Size: 8 GB, Speed: 3200 MHz Operating System: - Microsoft Windows 11 Home ( +Win32_OperatingSystem: Microsoft Windows 11 Home.+) |
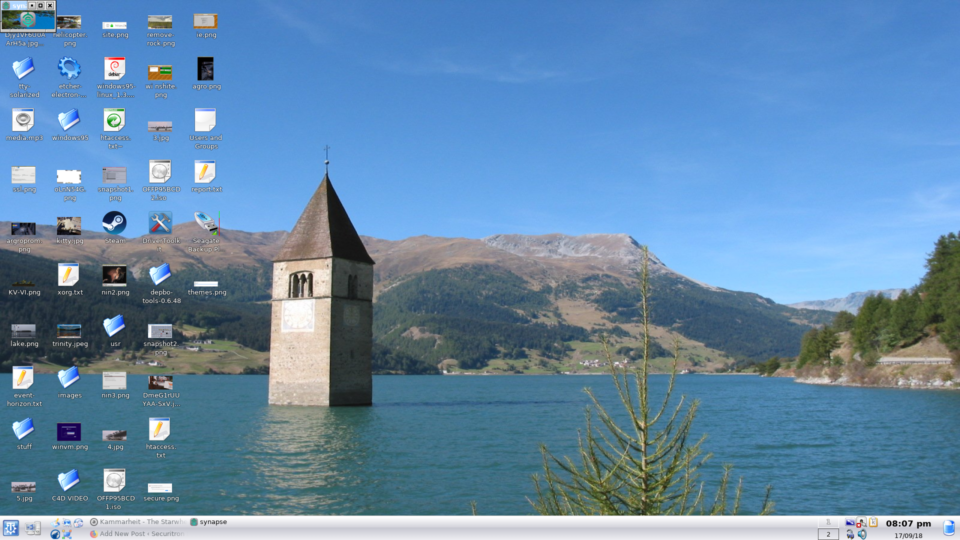
This should be a very useful script to print information about your computer hardware on Windows.
This version of the script will get the RAM speeds and capacity.
$SystemInfo = Get-CimInstance -ClassName Win32_ComputerSystem $CPUInfo = Get-CimInstance -ClassName Win32_Processor $MemoryInfo = Get-CimInstance -ClassName Win32_PhysicalMemory $OSInfo = Get-CimInstance -ClassName Win32_OperatingSystem Write-Host "Computer Name: $($SystemInfo.Name)" Write-Host "Manufacturer : $($SystemInfo.Manufacturer)" Write-Host "Model : $($SystemInfo.Model)" Write-Host "" Write-Host "CPU Information" foreach ($CPU in $CPUinfo) { Write-Host "- $($CPU.Name), Max Clock Speed: $($CPU.MaxClockSpeed) MHz" } Write-Host "" # Display Memory (RAM) information. Write-host 'Memory Information (RAM):' foreach ($memory in $Memoryinfo) { $CapacityGB = [math]::Round($memory.Capacity / 1GB, 2) Write-host ("- Manufacturer: {0}, Model: {1}, Size: {2} GB, Speed: {3} MHz" -f ` $memory.Manufacturer, $memory.PartNumber.Trim(), ${CapacityGB}, $($memory.Speed)) } write-host '' # Display OS information. write-host 'Operating System:' write-host '- '$osinfo.caption,'('+$osinfo.OSArchitecture+')' |
This will give you this output.
Computer Name: DESKTOP-PF01IEE Manufacturer : ASUS Model : System Product Name CPU Information - Intel(R) Core(TM) i5-10400F CPU @ 2.90GHz, Max Clock Speed: 2904 MHz Memory Information (RAM): - Manufacturer: Team Group Inc, Model: TEAMGROUP-UD4-3200, Size: 8 GB, Speed: 3200 MHz - Manufacturer: Team Group Inc, Model: TEAMGROUP-UD4-3200, Size: 8 GB, Speed: 3200 MHz Operating System: - Microsoft Windows 11 Home ( +Win32_OperatingSystem: Microsoft Windows 11 Home.OSArchitecture+) |
And to list all hard drives and the SSD drives in your machine, use this simple one-liner.
PS C:\Users\Intel i5> Get-PhysicalDisk | Select-Object DeviceId, Model, Name, MediaType, @{Name='Size (GB)'; Expression={$_.Size / 1GB -as [int]}} | Format-Table -AutoSize DeviceId Model Name MediaType Size (GB) -------- ----- ---- --------- --------- 0 CT1000BX500SSD1 SSD 932 1 CT500P2SSD8 SSD 466 2 Expansion Desk Unspecified 2795 3 My Passport 25E1 HDD 931 |
And this better version.
PS C:\Users\Intel i5> Get-PhysicalDisk | Select-Object DeviceId, Model, Name, MediaType, @{Name='Size (GB)'; Expression={$_.Size / 1GB -as [int]}},HealthStatus,SerialNumber | Format-Table -AutoSize DeviceId Model Name MediaType Size (GB) HealthStatus SerialNumber -------- ----- ---- --------- --------- ------------ ------------ 0 CT1000BX500SSD1 SSD 932 Healthy 2144E5E15CE3 1 CT500P2SSD8 SSD 466 Healthy 6479_A7FF_F000_0027. 2 Expansion Desk Unspecified 2795 Healthy NA8X6JZL 3 My Passport 25E1 HDD 931 Healthy WXS1A37KNS73 |